Stage 5: Game Lifecycle: drawing text, title screen, failure condition, and moving between levels
Currently, when monsters are killed they are immediately deleted from the game. That's great, but there is no code to handle
player death. Let's tackle that now.
Game state
We'll add the concept of a
so we can model the game as a
finite state machine with four states.
Our four states:
- loading: waiting for the assets to load
- title: on the title screen
- running: playing the game actively
- dead: the moment after the player has died, but before returning to the title screen
"Finite state machine" sounds super complicated. It's not.
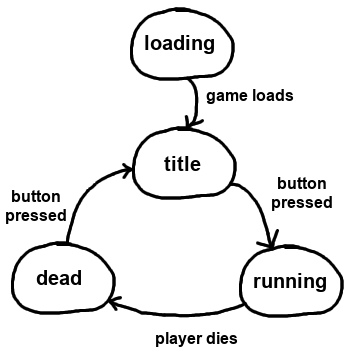
Just think about a title screen. That's a state. Then when the
actual game is running, that's a state. Clearly different things are displayed on the title screen versus the game proper. And pressing a specific button in-game and pressing it on the title screen do different things. So that's all we're trying to accomplish.
To make this work, we need to only do two things.
- When needed, switch states simply by setting to a different state name
- Use conditionals checking the value of to wrap behavior that should only occur in specific states
index.html
When our spritesheet image loads, we want to switch to showing the title screen which is accomplished by assigning a function to
(we'll write that
function below next). But what's our first state? Unsurprisingly, we're initializing
to "loading" because that's the first thing that happens.
While we're here, we're adding some variables related to the game lifecycle:
and
.
Within the
handler, you can see where we're starting to add transitions between game states when buttons are pressed and also restricting when gameplay actions can take place (only when "running").
Lastly, we're moving out some code related to setting the
location and generating a level. If we're going to have multiple levels, it no longer makes sense to do these things only once.
There are several small changes to add to game.js:
game.js
In
,
we restrict our draw operations to when the game is running OR when the player just died.
In
,
we change to the "dead" state when we detect that the player has died.
In
,
we draw a semi-transparent black background, which will function as our title screen for now. Then we change to the "title" state.
In
,
we jump to the first floor, call
, and we change to the "running" state.
In
,
we move over the code that we took out of index.html. Also
gets initalized with
equal to the value passed in
. This value starts as 3, but in a little bit we'll use this function to persist player HP across levels.
Now load up the game. You'll see the "title screen", a black box for now. When you hit a key, the game will start running. And if you were to die, everything would freeze until you pressed another key... leading back to the title screen, this time overlaid semitransparently over the game. That's the whole state machine right there working!
Spawning more monsters
It's not easy to die in this game. No monsters spawn after the first two. Let's make it harder.
game.js
We're using two new global variables.
-
counts down until each spawn and then resets
-
is how often monsters will get spawned and provides the initial value for every time it is reset
After every spawn,
is decremented so that monsters come out even faster.
After the first 15 turns, a new monster gets spawned
14 turns after that, another monster spawns
13 turns after that, another monster spawns
...
And so on until monsters spawn every turn
Tweaking the initial value of
will have a massive impact on the game's design (try a value of 1 for some real fun).
Try that out and see how you like the spawn behavior.
Teleport counter
One more rough edge to smooth out. Monsters can spawn right next to us and immediately start attacking. It's also very weird to see monsters spawning in the middle of the map with no explanation.
One convenient explanation is that these monsters are being "teleported" into the map. While each monster is teleporting, which will take one extra turn, they will be unable to do anything. Think of this as equivalent to Magic's "summoning sickness".
To visually demonstrate this, we need a teleporting sprite. Let's draw that before getting to the code.
Drawing the teleport sprite
There's not much to this one. We draw a spiral, add swirly limbs, and outline it.
To integrate this teleporting thing, we'll use a counter just like we did with
.
This should look familiar. You can do a
hell of a lot of work with only boolean flags for statuses and integer counters for longer lasting effects.
monster.js
The monsters are teleporting in and spawning nicely... eventually leading to an unwillable scenario. Let's give the player an
exit from this situation.
Drawing the exit sprite
I tried drawing something resembling a portal.
Our
tile is a pretty basic object, but it does need to do something (i.e. go to the next level) whenever the player moves onto it. It's within the monster code that we determine when we're stepping on something, but after that point it's best to delegate to the tiles themselves. Each tile will know exactly what to do when stepped on.
tile.js
Notice that we need an empty
method for
too because monsters will be stepping on them as well. It won't do anything for now but it's nice to leave a little reminder comment to finish up the behavior later. We'll never need this method for a
because monsters don't step on those. And unlike in other languages we don't need this method on the base object in order to call it on the subclasses.
Now what happens when the player steps on the
? If we were already on the last level, we jump to the title screen. Otherwise, we start a new level with 1 extra HP.
Two more pieces to tie it all together: triggering
and creating the
tile.
monster.js
game.js
With these small additions, the game has some real structure to it.
Title time
The title screen is seriously lacking though. I don't want to do anything too crazy for the title screen, but at the very least it needs some text.
If you're trying to draw UI elements for a browser game, I would typically recommend trying it with HTML. You've got a lot of functionality out of the box with HTML & CSS, built up over decades. But for a few simple lines of text, the canvas will do just fine.
Drawing text on the canvas is not much different from drawing images. The important bit here is at the end:
.
All we need to pass is what text to draw and where.
game.js
The first two lines of our function set the font color and size. The string
might look a little cryptic. We're actually writing a bit of CSS : we specify a font size in "px" units (pixels) and specify the type of font we want, which is "monospace".
We're going to leave the Y position up to the caller, but to make things easier this function will handle X. We're going to draw text in two places basically: justified centered on the title screen and on the far right (where the UI is) during play. The
variable lets us toggle between these two.
So what does this line mean exactly?
textX = (canvas.width-ctx.measureText(text).width)/2;
When you want to center
any element, whether on the canvas or in HTML, the calculation is the same. For X: half the container width minus half the content width. For Y: half the container height minus half the content height. The reason is obvious when you see it:
This is a good example of where HTML & CSS would be a lot easier to use! For one there are many easy ways to center things in CSS (there weren't for about 20 years but now there certainly are). And for two getting the width or height of an HTML element is very easy. None of this
nonsense. Did you know there isn't even a way to measure the height? Ugh!
Anyway, enough ranting... let's draw some text.
game.js
To review the arguments to our
function
- some text
- a font size
- whether the text is centered
- the Y position
- the color
Whew! That's a mouthful, but trust me when I say it's preventing us from duplicating a lot of code.
We're drawing the level number on the UI during play and drawing the name of the game on the title screen. I chose a silly name. I'm sure you can do better.
Try it out. I think it's looking good. At this point, the game can be considered a fully playable and complete game. It's just not very interesting yet. We'll get there.
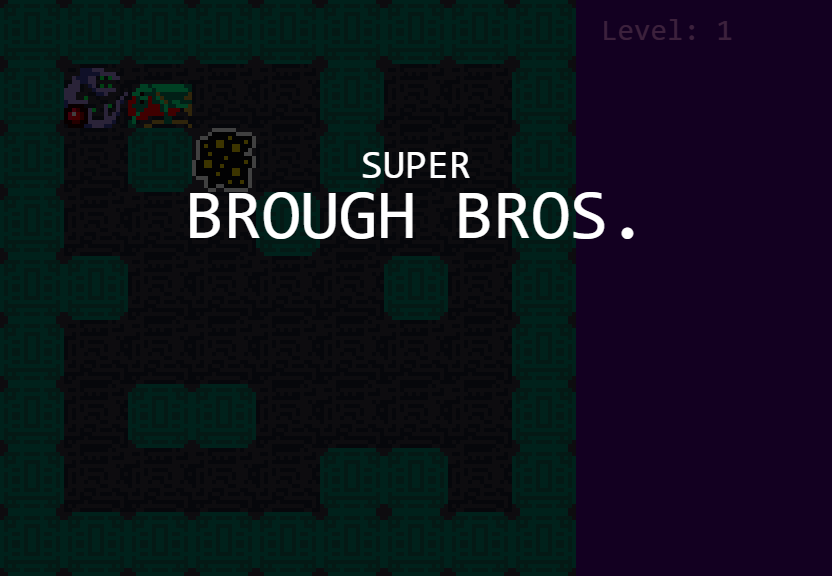
In the
next section, we'll be adding treasure and score mechanics.